Purebasic Serial Port Example
I am not looking for specific instructions here, just trying to find sources to research on how to proceed. I would like to take numbers from a Program written in purebasic and deliver them to a midi interface through the USB port. What I don't understand is how I tell the program to output a number to the USB port. For example your application can only access serial ports from 1 to 10 and Windows assigned your new serial port a number of 11. Well there is a way around it. Go to Control Panel - System - Hardware-Device Manager - Port - Properties-Advanced and fill in the COM number that you would like to have.
- Purebasic Serial Port Example List
- Purebasic Serial Port Example 1
- Purebasic Serial Port Example Free
- Purebasic Serial Port Examples
This page describes how to use the built-in serial ports (also knownas USARTs). For more information about serial ports, seeUSART.
Purebasic Serial Port Example List
Contents One hour one life crafting.
First, decide which serial port you wish to use, and connect itspins to the device you’re communicating with. (The TXand RX pins for a serial port are labeled on your board’s silkscreen;for example, serial port 2 has pins labeled “TX2” and “RX2”).
The variable for controlling a serial port is the word Serial
,plus the serial port’s number. For example, you can control serialport 1 with the variable Serial1
, serial port 2 with Serial2
,and so on.
In order to get started using your serial port, you’ll first need toturn it on. Do this by calling your serial port’s begin()
function, giving it the baud rate you wish it to communicate at. Ifyou’re not sure what baud rate to use, 9600 is a safe (although slow)value to try. Put this call to begin()
in your setup(),like in the following example:
Now that your serial port is set up, it’s time to start communicating.
One common use for serial ports is to print strings and otherdebugging information to a computer. You can print numbers or stringsusing print()
and println()
, like this:
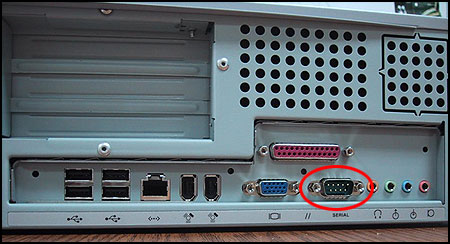
This sort of communication can go both ways: you can send charactersfrom a computer to a serial port as well. You can check how manycharacters are waiting for you to read using the available()
function, and read them out one at a time using read()
. Thefollowing example program uses these functions to “echo” back anythingsent to Serial2
:
This section gives a full listing of functions available for use withserial ports.
All of the Serial[1,2,3]
objects are instances of theHardwareSerial
class, which is documented in this section. (Thismeans that you can use any of these functions on any of Serial1
,Serial2
, and Serial3
).
HardwareSerial
¶Serial port class. Predefined instances are Serial1
,Serial2
, and Serial3
.
HardwareSerial::
begin
(unsigned int baud)¶Set up a HardwareSerial
object for communications. This methodmust be called before attempting to use the HardwareSerial
object (typically, you call this in your setup() function).
HardwareSerial::
end
()¶Disables the USART associated with this object, allowing anyassociated communication pins to be used for other purposes.
HardwareSerial::
available
()¶Returns the number of bytes available for reading.
unsigned char HardwareSerial::read()
Returns the next available, unread character. If there are noavailable characters (you can check this with available
), the call will block until onebecomes available.
HardwareSerial::
flush
()¶Throw away the contents of the serial port’s receiver (RX) buffer.That is, clears any buffered characters, so that the next characterread is guaranteed to be new.
void HardwareSerial::print(unsigned char b)
Print the given byte over the USART.
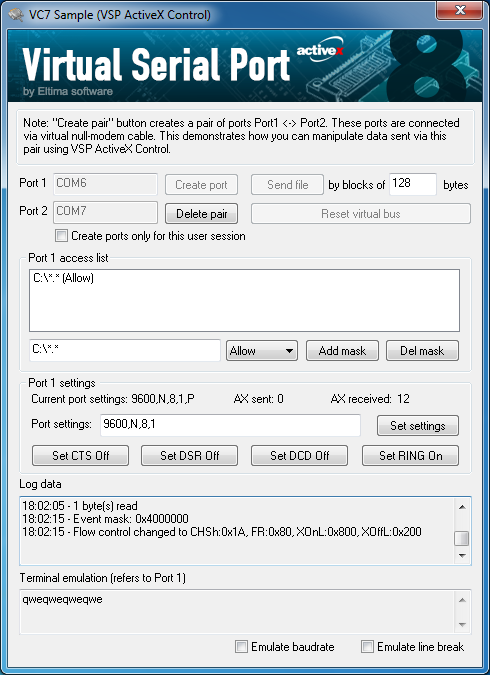
HardwareSerial::
print
(char c)¶Print the given character over the USART. 7-bit clean charactersare typically interpreted as ASCII text.
HardwareSerial::
print
(const char *str)¶Print the given null-terminated string over the USART.
HardwareSerial::
print
(int n)¶Print the argument’s digits over the USART, in decimal format.Negative values will be prefixed with a '-'
character.
HardwareSerial::
print
(unsigned int n)¶Print the argument’s digits over the USART, in decimal format.
HardwareSerial::
print
(long n)¶Print the argument’s digits over the USART, in decimal format.Negative values will be prefixed with a '-'
character. New cisco ios 15 license key generator 2016 free and reviews 2016 nissan.
HardwareSerial::
print
(unsigned long n)¶Print the argument’s digits over the USART, in decimal format.
HardwareSerial::
print
(long n, int base)¶Print the digits of n
over the USART, in base base
(whichmay be between 2 and 16). The base
value 2 corresponds tobinary, 8 to octal, 10 to decimal, and 16 to hexadecimal. Negativevalues will be prefixed with a '-'
character.
HardwareSerial::
print
(double n)¶Print n
, accurate to 2 digits after the decimal point.
HardwareSerial::
println
(char c)¶Like print(c)
, followed by 'rn'
.
HardwareSerial::
println
(const char *c)¶Like print(c)
, followed by 'rn'
.
void HardwareSerial::println(unsigned char b)
Like print(b)
, followed by 'rn'
.
HardwareSerial::
println
(int n)¶Like print(n)
, followed by 'rn'
.
HardwareSerial::
println
(unsigned int n)¶Like print(n)
, followed by 'rn'
.
HardwareSerial::
println
(long n)¶Like print(n)
, followed by 'rn'
.
HardwareSerial::
println
(unsigned long n)¶Like print(n)
, followed by 'rn'
.
HardwareSerial::
println
(long n, int base)¶Like print(n,b)
, followed by 'rn'
.
HardwareSerial::
println
(double n)¶Like print(n)
, followed by 'rn'
.
HardwareSerial::
println
()¶Prints 'rn'
over the USART.
void HardwareSerial::write(unsigned char ch)
Sends one character over the USART. This function is currentlyblocking.
This is a low-level function. One of the print()
orprintln()
functions is likely to be more useful when printingmultiple characters, when formatting numbers for printing, etc.
HardwareSerial::
write
(const char *str)¶Send the given null-terminated character string over the USART.
This is a low-level function. One of the print()
orprintln()
functions is likely to be more useful when printingmultiple characters, when formatting numbers for printing, etc.
HardwareSerial::
write
(void *buf, unsigned int size)¶Writes the first size
bytes of buf
over the USART. Eachbyte is transmitted as an individual character.
This is a low-level function. One of the print()
orprintln()
functions is likely to be more useful when printingmultiple characters, when formatting numbers for printing, etc.
HardwareSerial::
txPin
()¶Return the number of the TX (transmit) pin.
HardwareSerial::
rxPin
()¶Return the number of the RX (receive) pin.
Unlike the Arduino, none of the Maple’s serial ports is connected tothe USB port on the Maple board. If you want to communicate using thebuilt-in USB port, use SerialUSB instead. Youwill need an additional USB-to-serial adapter to communicate between aUSART and your computer.
License and Attribution
Portions of this page were adapted from the Arduino ReferenceDocumentation, whichis released under a Creative Commons Attribution-ShareAlike 3.0License.
Daycare Nightmare 2 Mini Monsters is the second edition of this series. Once again, the protagonist is a young woman in the babysitting business who’s obviously not afraid of monsters, since the special thing of her business is that she takes care of baby monsters instead of baby humans. There is a brief introduction to the game that tells you that many monsters are moving in to the city.
Purebasic Serial Port Example 1
Sep 19, 2017 - We are also considering not buying an apple product due to this. I did take pics of the laptop before I sent it out including the serial number.
Apparently it’s because the local witch has casted a charm over humans that make them see monsters as regular people. So they can lived in peace in this town. Your goal on the game is to help our young protagonist to take good care of the baby monsters and keep her on business. So let’s know more details about the game.
You have to take care of babies needs which go in a specific cycle. Anticipate the next need of a baby to fulfill it faster. If a baby completes a cycle of needs without loosing happiness it will earn a halo. The Happy Meter fills when your babies are happy, and empties when they are upset. If the Happy Meter ever empties completely, you will lose the level.
Halos provide a bonus to tips and happiness. You can increase the bonus by completing further cycles of needs without the baby losing happiness. There a color code for the bonuses: Yellow X2, Red X3, Blue X4, and Purple X5.
Parents pick up and drop off their babies at the door. Click the door to receive them or hand them off. If babies are happy when you return them to their parents, you will receive a bigger tip. When little ones get tired, place them in a crib for a rap. Put hungry babies in a high chair to be fed.
After a fight, both babies need band-aids. Take the baby to a first aid station to fix their boo boo, or keep a band-aid for emergencies. Place bored babies on a playmat to keep them entertained. When a baby wets itself, take it to a changing table to change it, or keep a diaper on hand for emergencies. Place babies on the carpet to increase their happiness over time. While on the carpet babies may fight or play with each other.
Purebasic Serial Port Example Free
There are several types of babies, or better said, baby moods. So you have to keep an eye on the differences and special needs of each type of baby so you can keep them all happy. Between levels you can visit the curiosity shop where you can spend your tip money to purchase new items to add to your daycare. Or upgrade pieces of furniture to let you more efficiently care of babies.
There you will find items to help you with baby needs or special items that give you some much needed help during here hectic day. When you first enter the game, you’ll see the welcome screen with the following options: - Change Player: the first time there’s no existing player. After you create one or more, they’ll be saved. When you enter into this submenu, you’ll be asked for a name as a new player. It’s possible to have a list of different players and record their performance. This way, you can play home tournaments with family and friends. - Play: the game introduction will be shown but you can skip at any time.
Then you’ll be seeing the tutorial to learn the basics. - Options: this is the menu from which you can set some game options like music and sound volume, full screen, show tutorial, and credits. - Help: from here you can access some help information to know the basics. - Exit: no further explanation required. You can try this game for 60 minutes before you buy the licensed version.
Seller Inventory # 4. Risunki protiv kureniya. Condition: Good + to Very Good. Pages: 640 EAN 029. To United Kingdom About this Item: Sovetskii Pisatel', Moscow, 1967.
Learning Laravel Laravel has the most extensive and thorough documentation and video tutorial library of any modern web application framework. The is thorough, complete, and makes it a breeze to get started learning the framework. A superb combination of simplicity, elegance, and innovation give you tools you need to build any application with which you are tasked.
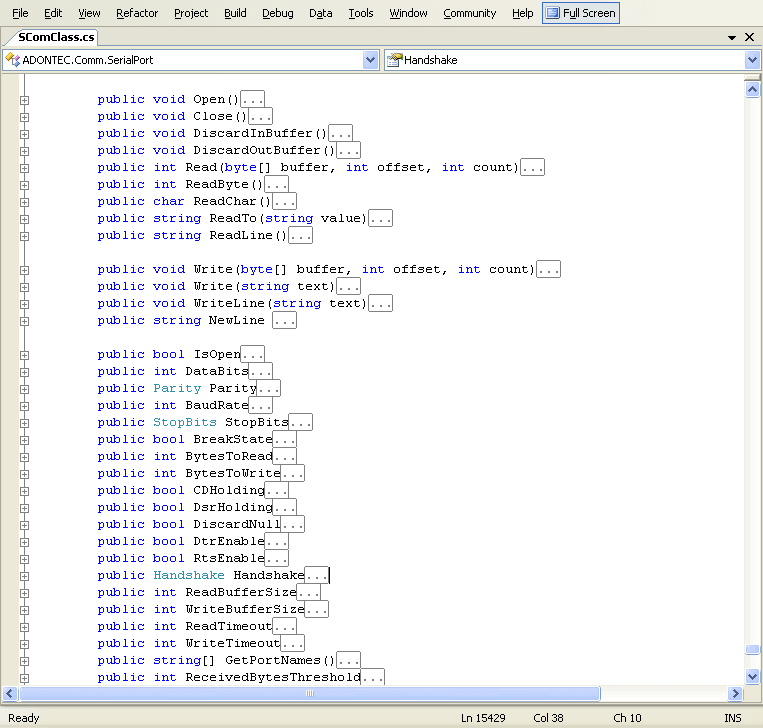
Purebasic Serial Port Examples
If you like it, you can buy this game for only 19.95 dollars, right from the Oberon Media Game Center, on the Web. About the systems requirements, here you have a list to check out: • OS: Windows® XP/Vista • Processor: Pentium® III 700 MHz • RAM: 128 MB • Minimum Screen Resolution: 800x600 • Sound Card: recommended • DirectX® Version: DirectX 7.0 • Hard Drive Space: 30MB File Distribution Notice of Daycare Nightmare Mini Monsters Shareware - Daycare Nightmare Mini Monsters Free Download - 2000 Shareware periodically updates software information of Daycare Nightmare Mini Monsters from the publisher, so some information may be slightly out-of-date.